Hi,
I want to play continuous sequences on the Fastino, where each channel gets updated at the same time with the highest possible sampling rate. My minimal code example for that looks like the following:
(Very similar to the example in the documentation here: https://m-labs.hk/artiq/manual/getting_started_core.html#direct-memory-access-dma)
from artiq.experiment import *
from artiq.language.environment import EnvExperiment
from artiq.frontend import artiq_run
from artiq.language.core import (delay, kernel, )
from artiq.language.units import ms
class Fastino_Test(EnvExperiment):
def build(self):
self.setattr_device("core")
self.setattr_device("core_dma")
self.setattr_device("dev_fastino0")
self.data_1 = [1 for _ in range(0, 32)]
self.data_2 = [2 for _ in range(0, 32)]
@kernel
def record(self):
with self.core_dma.record("test_sequence"):
for _ in range(0, 100):
self.dev_fastino0.set_group(0, self.data_1)
delay(10 * ms)
self.dev_fastino0.set_group(0, self.data_2)
delay(10 * ms)
self.dev_fastino0.set_group(0, self.data_1)
delay(10 * ms)
self.dev_fastino0.set_group(0, self.data_2)
delay(10 * ms)
@kernel
def run(self):
self.core.reset()
self.record()
test_sequence = self.core_dma.get_handle("test_sequence")
self.core.break_realtime()
while True:
self.core_dma.playback_handle(test_sequence)
if __name__ == "__main__":
artiq_run.run()
pass
The corresponding output on our Oscilloscope looks like this:
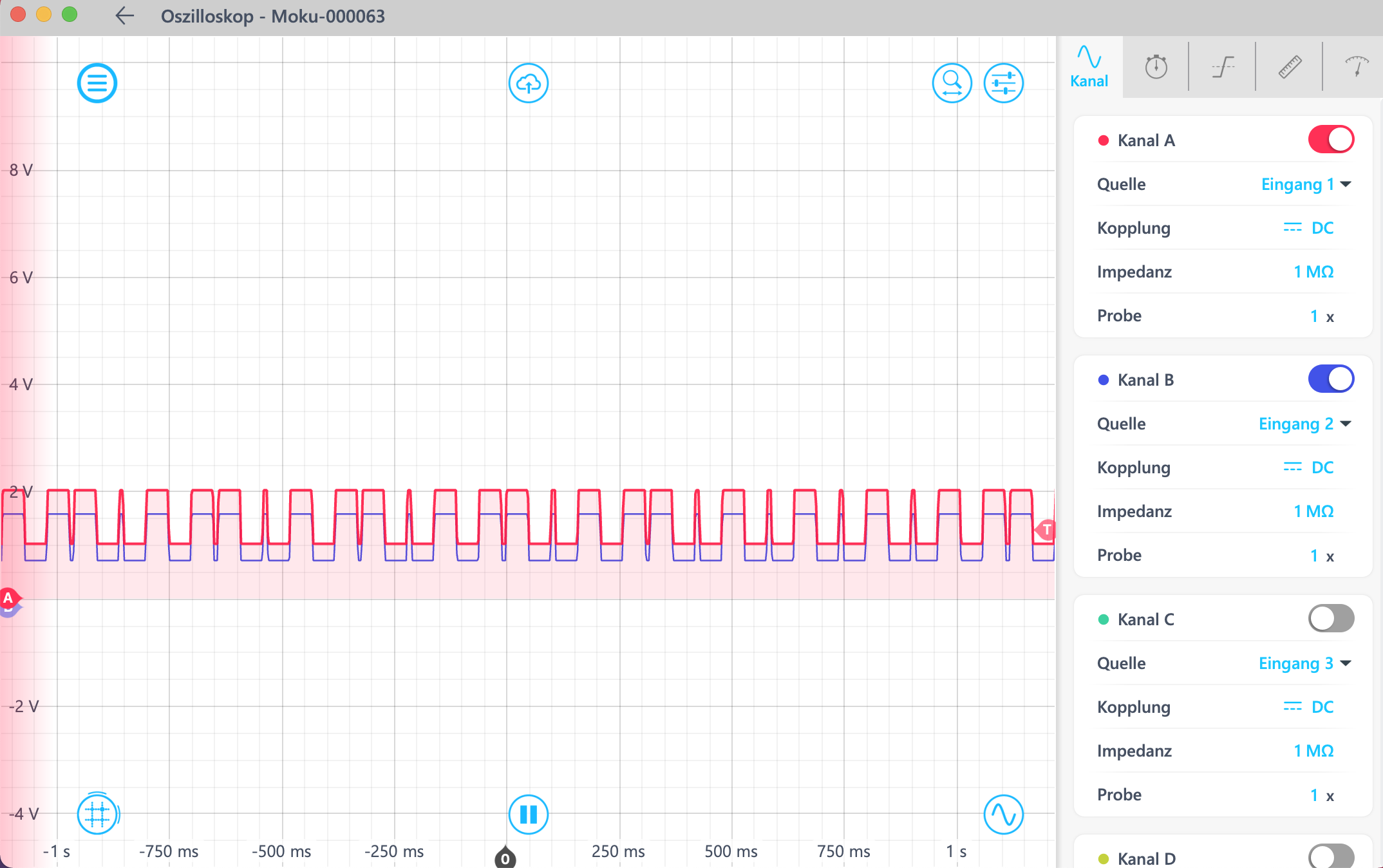
The pattern does not match the implementation, i.e. the delays are not equidistant and often too long.
I also tested the same pattern without DMA and everything looks fine.
Am I missing something or is there another way to achieve the desired output with DMA?