We are releasing ARTIQ-8 today, packed with new features and scalability and performance improvements.
DRTIO satellites are now able to run kernels ("subkernels") locally, in parallel with the main kernel. The API looks like this:
from artiq.experiment import *
class SubkernelExperiment(EnvExperiment):
def build(self):
self.setattr_device("core")
self.setattr_device("ttl0")
self.setattr_device("ttl8") # on satellite at destination 1
@subkernel(destination=1)
def add_and_pulse(self, a: TInt32, b: TInt32) -> TInt32:
c = a + b
self.pulse_ttl(c)
return c
@subkernel(destination=1)
def pulse_ttl(self, delay: TInt32) -> TNone:
self.ttl8.pulse(delay*us)
@kernel
def run(self):
subkernel_preload(self.add_and_pulse)
self.core.reset()
delay(10*ms)
self.add_and_pulse(2, 2)
self.ttl0.pulse(15*us)
result = subkernel_await(self.add_and_pulse)
assert result == 4
self.pulse_ttl(20)
Besides arguments and return values, subkernels can also pass messages between each other or the main kernel with the built-in subkernel_send()
and subkernel_recv()
functions. This can be used for communication between subkernels, passing additional data, or partially computed data.
Heterogenous systems mixing RISC-V devices (e.g. Kasli) and Arm devices (e.g. Kasli-SoC) are supported.
DMA playback can now be distributed using the local SDRAM memory of each satellite, for increased throughput and scalability.
On the GUI side, the monitoring/injection system has been revamped and only user-specified channels are displayed, so that systems with large numbers of (D)RTIO channels can be managed. It is also possible to open multiple monitoring/injection panels and reorganize arbitrary widgets between them, using the GUI.
Many other user interface features and improvements have landed in ARTIQ-8. The RTIO analyzer is now integrated with the dashboard, removing the need for external VCD viewers such as GtkWave:
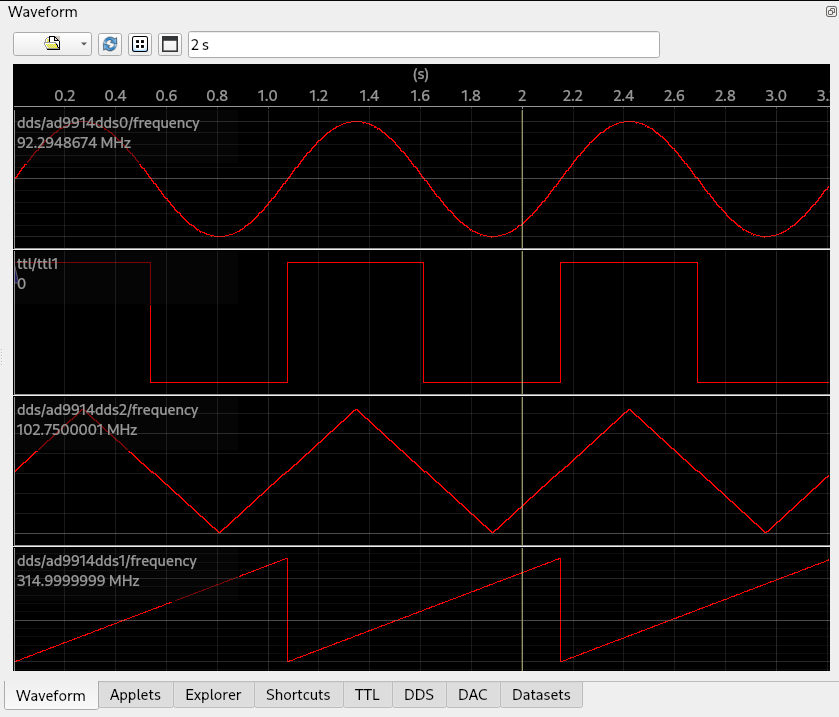
Experiments can now request user input at any time during their execution.
with self.interactive(title="Request_1") as sc2:
sc2.setattr_argument("sc2_boolean", BooleanValue(False),
"Transporter")
sc2.setattr_argument("sc2_scan", Scannable(default=RangeScan(200, 300, 49)),
"Transporter")
sc2.setattr_argument("sc2_enum", EnumerationValue(["3", "4", "5"]),
"Transporter")
The request would be displayed in the dashboard as follows:
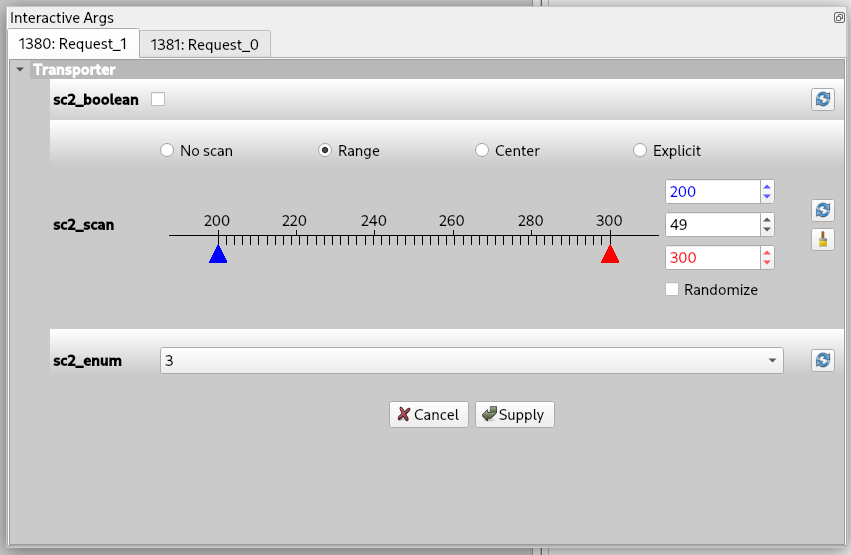
Datasets can have units associated with them:
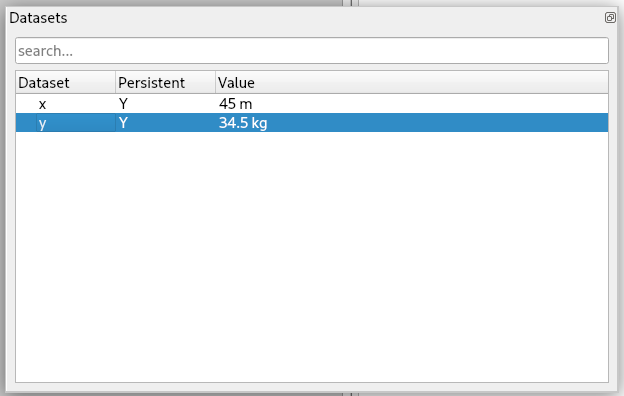
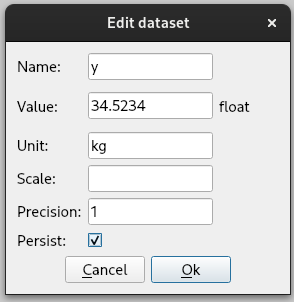
Applets can use experiment entry widgets:
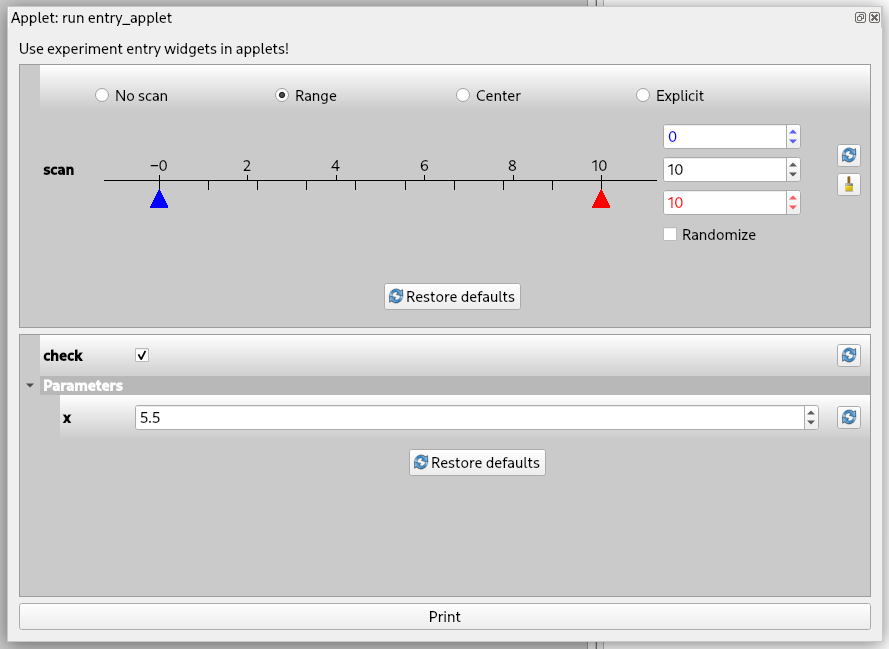
Applets can now also modify datasets in a simple way and without requiring an additional user-defined connection to the master.
On the hardware side, another exciting feature is support for the Sinara EFC/Shuttler 16-channel 125MSPS 14-bit DAC, with on the gateware side an upgraded version of the NIST PDQ-style waveform synthesizer (https://arxiv.org/abs/1301.2543) which also supports sigma-delta modulation to increase the effective bit depth. The Shuttler is connected to a remote analog front-end with +/-10V output and 50MHz 3dB bandwidth, with integrated 24-bit ADC for calibration.
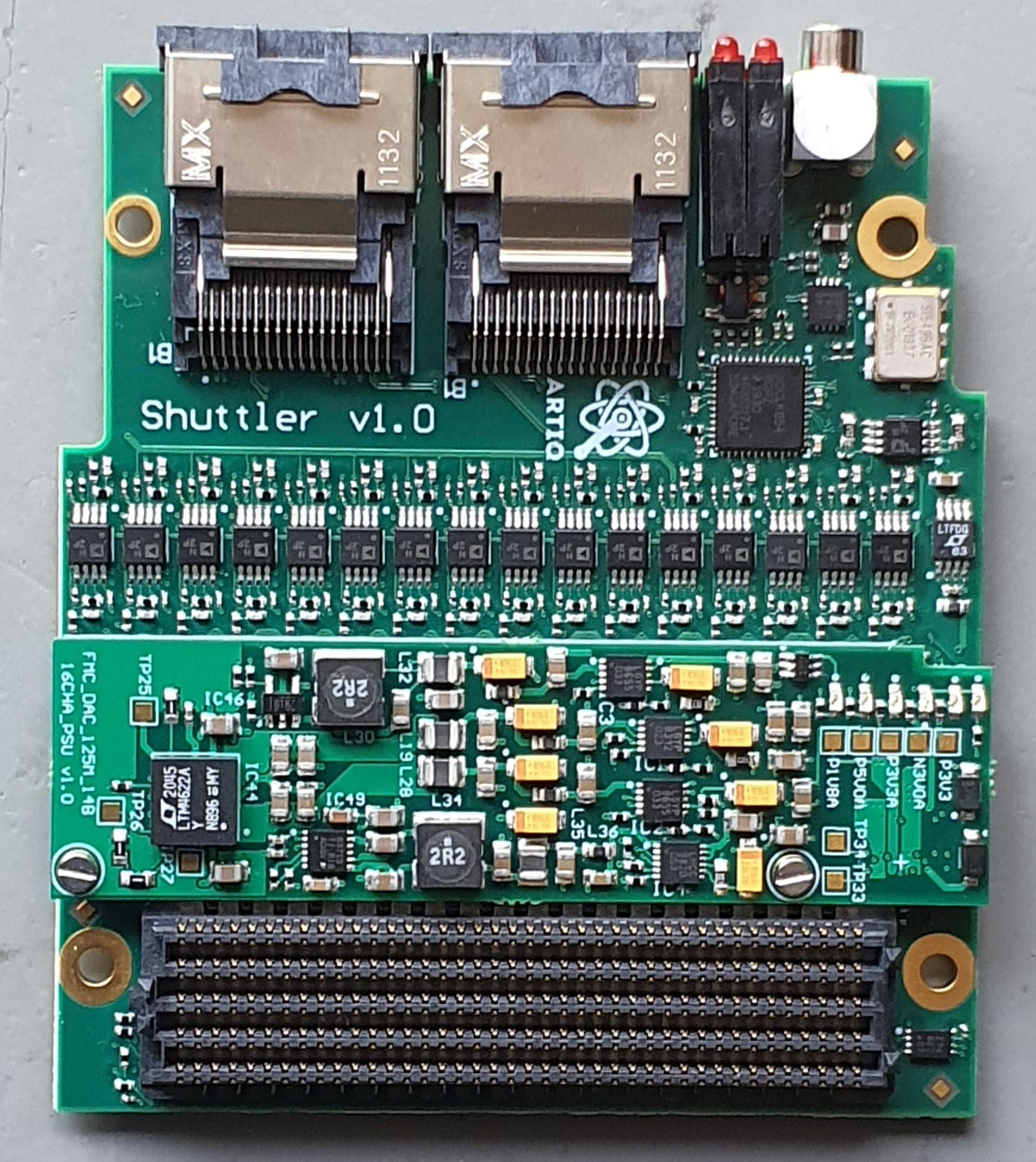
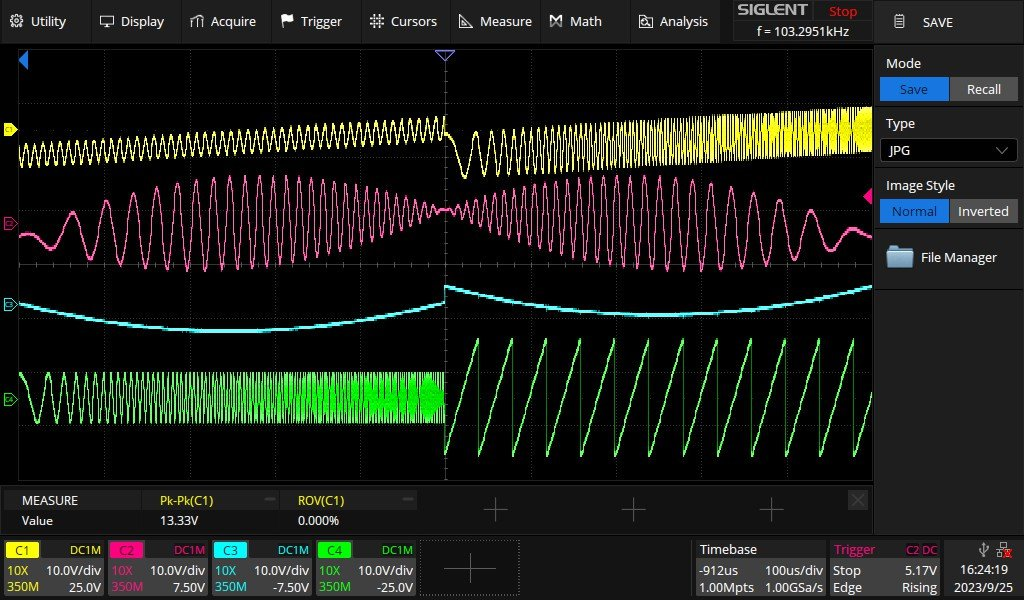
ARTIQ-8 supports DRTIO-over-EEM and EFC/Shuttler is the first peripheral to make use of it. This means that subkernels and distributed DMA, as well as any future DRTIO improvements, can be used on EFC/Shuttler. Sinara Phaser should follow suit in a future ARTIQ version.
Another major DRTIO improvement in ARTIQ-8 is the implementation of WRPLL clock recovery, based on CERN's White Rabbit Low Jitter design and using the Si549 low-noise oscillators on the Kasli 2.x and Kasli-SoC platforms. This results in lower noise than the Si5324-based clock recovery used in earlier ARTIQ versions, which should in many cases eliminate the need to distribute separately a low-noise clock for data converters on DRTIO satellites. The SiPhaser hack is also no longer necessary since, unlike the Si5324, WRPLL is designed for deterministic phase from the ground up. WRPLL can also be used without DRTIO to synthesize clocks from the reference supplied by the user on the SMA input, effectively rendering the Si5324 obsolete on the Kasli 2.x and Kasli-SoC platforms. WRPLL is currently an opt-in feature and should become the default in a future ARTIQ version.
ARTIQ-8 now uses LMDB to store the dataset database with better performance, resolving the master lags that users with large dataset databases would experience. The file format is not backwards compatible and legacy PYON databases should be converted to LMDB with the script below:
from sipyco import pyon
import lmdb
old = pyon.load_file("dataset_db.pyon")
new = lmdb.open("dataset_db.mdb", subdir=False, map_size=2**30)
with new.begin(write=True) as txn:
for key, value in old.items():
txn.put(key.encode(), pyon.encode((value, {})).encode())
new.close()
On the software packaging side, ARTIQ-8 introduces packages for Windows based on the MSYS2 platform. This is meant to replace Conda which has a number of technical and licensing issues. MSYS2 is a free software project which allows Windows applications to be built, packaged and distributed using only free software tools; it also facilitates building independent and offline setups whereas Conda tries to push users and developers into their proprietary cloud ecosystem.
To make ARTIQ easier to set up on air-gapped Windows machines or where internet connectivity is otherwise limited, ARTIQ-8 comes with a self-contained .exe installer which sets up MSYS2, ARTIQ and a small collection of useful packages, without requiring an internet connection. Linux installation (with Nix or Conda) still requires internet access.
The MSYS2 project accepts sponsors and donations (https://github.com/sponsors/msys2).
ARTIQ-8 now fully supports Python 3.11, in particular regarding its new asyncio APIs.
There are also a number of smaller additions as well as some breaking changes, see the release notes in the repository for more details:
https://github.com/m-labs/artiq/blob/release-8/RELEASE_NOTES.rst
To upgrade from ARTIQ-6 or ARTIQ-7, perform a new software installation according to the manual:
https://m-labs.hk/artiq/manual/installing.html
You will also need to update the firmware in the core devices. Firmware binaries for your system can be obtained from M-Labs through AFWS.
Unless otherwise noted, old devices such as those based on Kasli 1.x remain supported by ARTIQ-8.